Back to pre-built integrations
News API to email integration
Integrating news headlines directly into Gmail through the News API and email integration carries significant advantages in today's fast-paced information landscape. By seamlessly delivering relevant and timely news updates within the familiar interface of Gmail, users can effortlessly stay informed without the need to toggle between multiple platforms.
The example below demonstrates how to integrate the News API to receive an email containing BBC headlines.
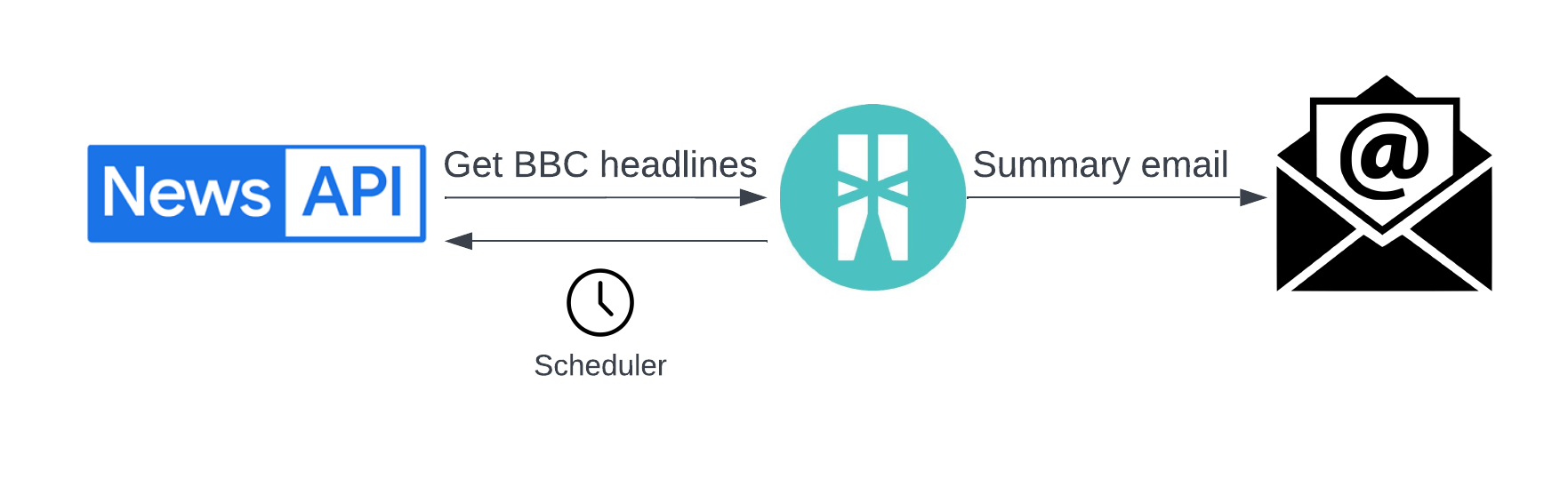
import ballerina/email;
import ballerina/log;
import ballerinax/newsapi;
// News API configuration
configurable newsapi:ApiKeysConfig apiKeyConfig = ?;
// Email client configuration parameters
configurable string smtpPassword = ?;
configurable string smtpUsername = ?;
configurable string smtpHost = ?;
// Email configuration parameters
configurable string fromAddress = ?;
configurable string emailAddress = ?;
public function main() returns error? {
newsapi:Client newsapi = check new (apiKeyConfig, {}, "https://newsapi.org/v2");
email:SmtpClient email = check new (smtpHost, smtpUsername, smtpPassword);
newsapi:WSNewsTopHeadlineResponse topHeadlines = check newsapi->listTopHeadlines(sources = "bbc-news", page = 1);
newsapi:WSNewsArticle[]? articles = topHeadlines?.articles;
if articles is () || articles.length() == 0 {
log:printInfo("No news found");
return;
}
string mailBody = "BBC top news are,
";
foreach newsapi:WSNewsArticle article in articles {
string? title = article?.title;
if title is string {
mailBody = mailBody + string `${title}${"
"}`;
}
}
email:Message message = {
to: emailAddress,
'from: fromAddress,
subject: "BBC Headlines",
body: mailBody
};
check email->sendMessage(message);
log:printInfo("Email sent successfully!");
}
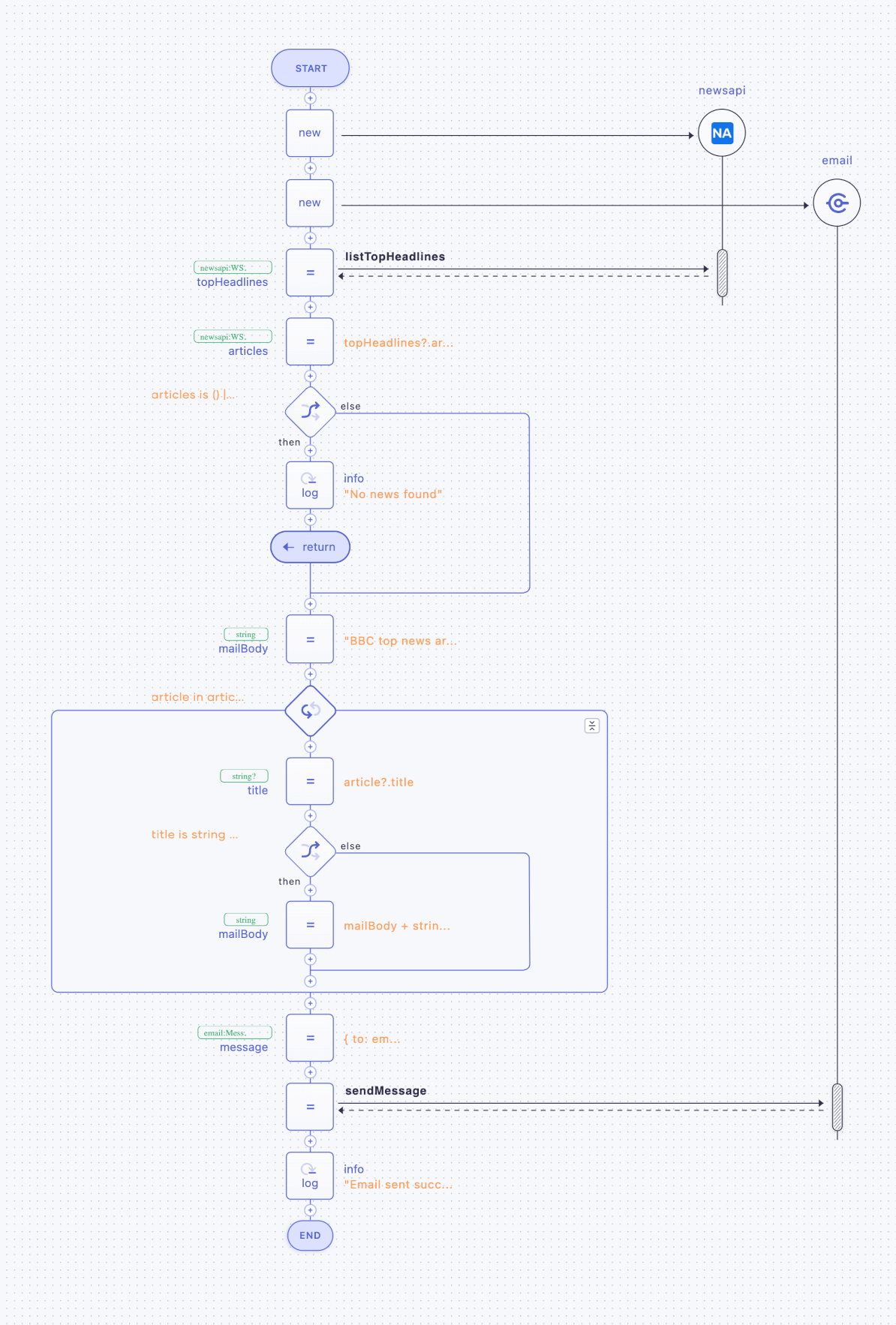